Floating Button Bot
Tin nhắn nhanh trong ứng dụng
Bạn sở hữu một trang thương mại điện tử và muốn khách hàng có thể tương tác trực tiếp với chat bot, CS team để giải quyết các băn khoăn khi mua hàng, giải quyết khiếu nại... Bubble chat bot có thể là một giải pháp cho bạn.
Dưới đây là 1 ví dụ để bạn mở cuộc hội thoại với Floating Button (sử dụng PSConversation).
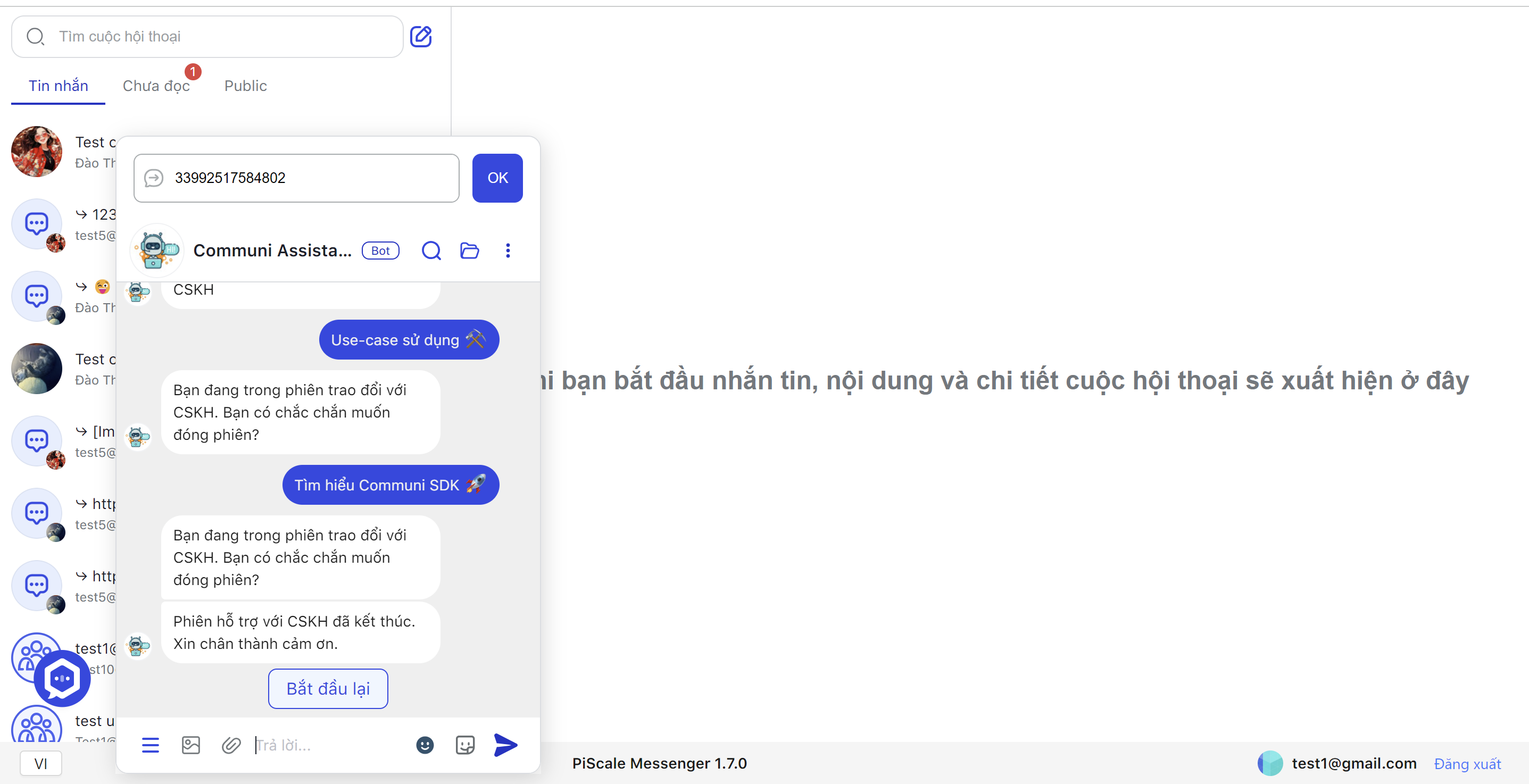
import { useState } from "react";
import { Popover } from "react-tiny-popover";
import {
PSChatProvider,
PSUIProvide,
PSConversation,
} from "@communi/chat-react";
export const App = () => {
const [isModalOpen, setIsModalOpen] = useState(false);
const [threadId, setThreadId] = useState(null);
const fetchCommuniToken = () => {
return "YOUR_COMMUNI_TOKEN";
};
return (
<PSChatProvider appId="YOUR_APP_ID" fetchToken={fetchCommuniToken}>
<Popover
isOpen={isModalOpen}
containerStyle={{ zIndex: 10 }}
content={
<PSUIProvider style={{ width: 400, height: 800 }}>
<PSConversation
threadId={threadId}
userId="YOUR_USER_ID_OR_BOT_ID"
onThreadChange={(threadId) => setThreadId(threadId)}
/>
</PSUIProvider>
}
>
<button
style={{
position: "absolute",
width: 40,
height: 40,
bottom: 60,
right: 30,
}}
onClick={() => setIsModalOpen(!isModalOpen)}
/>
</Popover>
</PSChatProvider>
);
};
Nguyên tắc để thực hiện việc này chính là hãy vẽ PSConversation
trên bất kì khung giao diện nào bạn muốn. Mọi việc còn lại sẽ do chúng tôi xử lý.
Trong ví dụ trên chúng tôi đang sử dụng package react-tiny-popover
để hiển thị lên một popover chứa PSConversation
Floating Button Unread Count
Sử dụng useSelectThread
trong hooks để hiển thị số lượng tin nhắn chưa đọc trên Floating Button.
import { useState } from "react";
import { Popover } from "react-tiny-popover";
import {
PSChatProvider,
PSUIProvide,
PSConversation,
PSHelper,
useIsInitSuccess,
} from "@communi/chat-react";
const UnreadCount = ({ threadId }) => {
const thread = useSelectThread(threadId);
return <span>{thread.unread_count}</span>;
};
const BubbleChatPopover = ({ threadId }) => {
const [isModalOpen, setIsModalOpen] = useState(false);
return (
<Popover
isOpen={isModalOpen}
containerStyle={{ zIndex: 10 }}
content={
<PSUIProvider style={{ width: 400, height: 800 }}>
<PSConversation
threadId={threadId}
onThreadChange={(threadId) => setThreadId(threadId)}
/>
</PSUIProvider>
}
>
<button
style={{
position: "absolute",
width: 40,
height: 40,
bottom: 60,
right: 30,
}}
onClick={() => setIsModalOpen(!isModalOpen)}
/>
<UnreadCount threadId={threadId} />
</Popover>
);
};
const BubbleChat = () => {
const [threadId, setThreadId] = useState(null);
const [isInitPSProviderSuccess, setIsInitPSProviderSuccess] = useState(false);
useEffect(() => {
if (!isInitPSProviderSuccess) return;
PSHelper.getDirectThreadIdByUserId("YOU_BOT_ID").then((id) =>
setThreadId(id)
);
}, [isInitPSProviderSuccess]);
return threadId ? <ChatBotPopover threadId={threadId} /> : null;
};
export const App = () => {
const fetchCommuniToken = () => {
return "YOUR_COMMUNI_TOKEN";
};
return (
<PSChatProvider
appId="YOUR_APP_ID"
fetchToken={fetchCommuniToken}
onInitSuccess={setIsInitPSProviderSuccess}
>
<BubbleChat />
</PSChatProvider>
);
};